Android Starting New Activity Without Registering Receiver Again
When you complete the previous lesson, y'all take an app that shows an activeness that consists of a single screen with a text field and a Send push button. In this lesson, you add some code to the MainActivity
that starts a new activity to display a message when the user taps the Transport button.
Reply to the Ship push
Follow these steps to add together a method to the MainActivity
class that's called when the Send button is tapped:
-
In the file app > java > com.case.myfirstapp > MainActivity, add together the following
sendMessage()
method stub:Kotlin
class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) } /** Chosen when the user taps the Ship push */ fun sendMessage(view: View) { // Practice something in response to push button } }
Java
public course MainActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); } /** Called when the user taps the Send push button */ public void sendMessage(View view) { // Do something in response to button } }
You might see an error because Android Studio cannot resolve the
View
class used as the method argument. To clear the error, click theView
proclamation, identify your cursor on information technology, and then press Alt+Enter, or Option+Enter on a Mac, to perform a Quick Set up. If a menu appears, select Import class. - Render to the activity_main.xml file to call the method from the button:
- Select the button in the Layout Editor.
- In the Attributes window, locate the onClick belongings and select sendMessage [MainActivity] from its drop-down list.
Now when the button is tapped, the organisation calls the
sendMessage()
method.Accept note of the details in this method. They're required for the organisation to recognize the method as compatible with the
android:onClick
aspect. Specifically, the method has the post-obit characteristics:- Public admission.
- A void or, in Kotlin, an implicit unit of measurement return value.
- A
View
every bit the only parameter. This is theView
object yous clicked at the cease of Step 1.
- Adjacent, fill in this method to read the contents of the text field and deliver that text to some other action.
Build an intent
An Intent
is an object that provides runtime binding betwixt split up components, such equally two activities. The Intent
represents an app'south intent to exercise something. Y'all can employ intents for a wide variety of tasks, but in this lesson, your intent starts another activity.
In MainActivity
, add the EXTRA_MESSAGE
constant and the sendMessage()
code, every bit shown:
Kotlin
const val EXTRA_MESSAGE = "com.instance.myfirstapp.Message" class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) } /** Called when the user taps the Ship button */ fun sendMessage(view: View) { val editText = findViewById<EditText>(R.id.editTextTextPersonName) val bulletin = editText.text.toString() val intent = Intent(this, DisplayMessageActivity::grade.java).apply { putExtra(EXTRA_MESSAGE, message) } startActivity(intent) } }
Java
public class MainActivity extends AppCompatActivity { public static final String EXTRA_MESSAGE = "com.example.myfirstapp.MESSAGE"; @Override protected void onCreate(Package savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); } /** Called when the user taps the Send push */ public void sendMessage(View view) { Intent intent = new Intent(this, DisplayMessageActivity.class); EditText editText = (EditText) findViewById(R.id.editTextTextPersonName); String message = editText.getText().toString(); intent.putExtra(EXTRA_MESSAGE, message); startActivity(intent); } }
Look Android Studio to run into Cannot resolve symbol errors once more. To clear the errors, press Alt+Enter, or Option+Return on a Mac. You should end upwards with the following imports:
Kotlin
import androidx.appcompat.app.AppCompatActivity import android.content.Intent import android.os.Bundle import android.view.View import android.widget.EditText
Java
import androidx.appcompat.app.AppCompatActivity; import android.content.Intent; import android.bone.Packet; import android.view.View; import android.widget.EditText;
An fault withal remains for DisplayMessageActivity
, but that'south okay. Y'all gear up information technology in the next section.
Here's what's going on in sendMessage()
:
-
The
Intent
constructor takes two parameters, aContext
and aCourse
.The
Context
parameter is used first because theActiveness
class is a subclass ofContext
.The
Class
parameter of the app component, to which the system delivers theIntent,
is, in this case, the activity to start. -
The
putExtra()
method adds the value ofEditText
to the intent. AnIntent
tin bear data types every bit primal-value pairs called extras.Your key is a public abiding
EXTRA_MESSAGE
considering the next activeness uses the key to call up the text value. It'southward a adept practice to ascertain keys for intent extras with your app's parcel name as a prefix. This ensures that the keys are unique, in example your app interacts with other apps. - The
startActivity()
method starts an example of theDisplayMessageActivity
that's specified past theIntent
. Side by side, yous need to create that grade.
Create the second activity
To create the second activity, follow these steps:
- In the Project window, correct-click the app binder and select New > Activity > Empty Activity.
- In the Configure Action window, enter "DisplayMessageActivity" for Activity Proper noun. Go out all other properties set to their defaults and click Stop.
Android Studio automatically does iii things:
- Creates the
DisplayMessageActivity
file. - Creates the layout file
activity_display_message.xml
, which corresponds with theDisplayMessageActivity
file. - Adds the required
<activity>
element inAndroidManifest.xml
.
If you run the app and tap the push button on the starting time activity, the 2d activity starts merely is empty. This is because the second activity uses the empty layout provided by the template.
Add together a text view
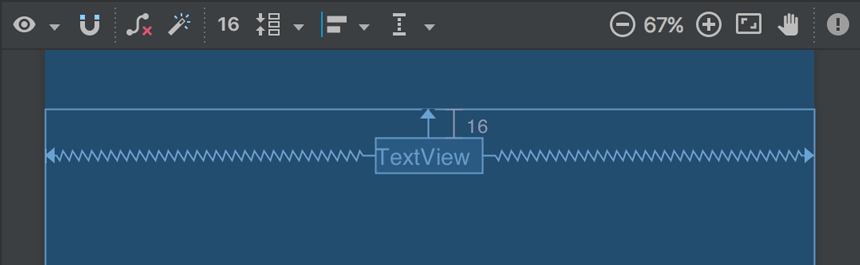
The new activeness includes a blank layout file. Follow these steps to add a text view to where the message appears:
- Open up the file app > res > layout > activity_display_message.xml.
- Click Enable Autoconnection to Parent
in the toolbar. This enables Autoconnect. See figure ane.
- In the Palette panel, click Text, drag a TextView into the layout, and drib it about the top-center of the layout so that it snaps to the vertical line that appears. Autoconnect adds left and right constraints in order to identify the view in the horizontal center.
- Create 1 more constraint from the top of the text view to the top of the layout, so that it appears as shown in effigy ane.
Optionally, you can make some adjustments to the text style if you expand textAppearance in the Mutual Attributes panel of the Attributes window, and change attributes such as textSize and textColor.
Display the message
In this stride, you modify the second activity to brandish the message that was passed by the start activity.
-
In
DisplayMessageActivity
, add the following code to theonCreate()
method:Kotlin
override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_display_message) // Get the Intent that started this activeness and extract the string val bulletin = intent.getStringExtra(EXTRA_MESSAGE) // Capture the layout's TextView and set up the cord as its text val textView = findViewById<TextView>(R.id.textView).utilize { text = bulletin } }
Java
@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_display_message); // Go the Intent that started this activity and extract the string Intent intent = getIntent(); String bulletin = intent.getStringExtra(MainActivity.EXTRA_MESSAGE); // Capture the layout's TextView and set the string equally its text TextView textView = findViewById(R.id.textView); textView.setText(message); }
-
Press Alt+Enter, or Choice+Return on a Mac, to import these other needed classes:
Kotlin
import androidx.appcompat.app.AppCompatActivity import android.content.Intent import android.os.Packet import android.widget.TextView
Coffee
import androidx.appcompat.app.AppCompatActivity; import android.content.Intent; import android.os.Bundle; import android.widget.TextView;
Add upward navigation
Each screen in your app that's non the master entry betoken, which are all the screens that aren't the dwelling screen, must provide navigation that directs the user to the logical parent screen in the app'due south hierarchy. To practice this, add an Up button in the app bar.
To add together an Up button, you need to declare which activity is the logical parent in the AndroidManifest.xml
file. Open the file at app > manifests > AndroidManifest.xml, locate the <action>
tag for DisplayMessageActivity
, and replace it with the following:
<activity android:name=".DisplayMessageActivity" android:parentActivityName=".MainActivity"> <!-- The meta-information tag is required if you support API level fifteen and lower --> <meta-information android:name="android.back up.PARENT_ACTIVITY" android:value=".MainActivity" /> </action>
The Android system now automatically adds the Upward push to the app bar.
Run the app
Click Utilize Changes in the toolbar to run the app. When information technology opens, blazon a bulletin in the text field and tap Send to see the message announced in the second activity.
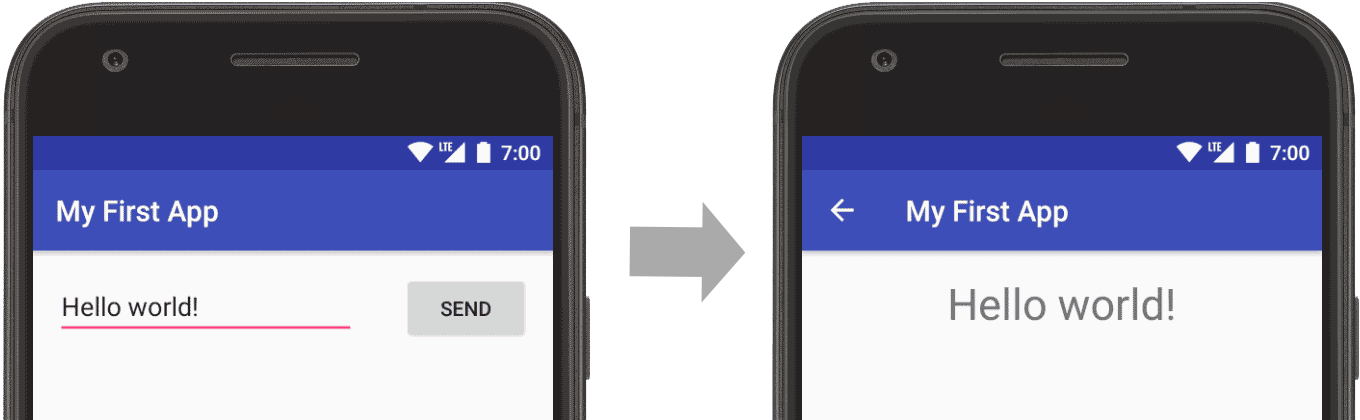
That'south it, you've built your first Android app!
To continue to learn the basics nigh Android app development, go back to Build your first app and follow the other links provided at that place.
Source: https://developer.android.com/training/basics/firstapp/starting-activity
0 Response to "Android Starting New Activity Without Registering Receiver Again"
Post a Comment